The Documentation Paradox: Why Better Docs Sometimes Lead to Worse Code (And How to Fix It)
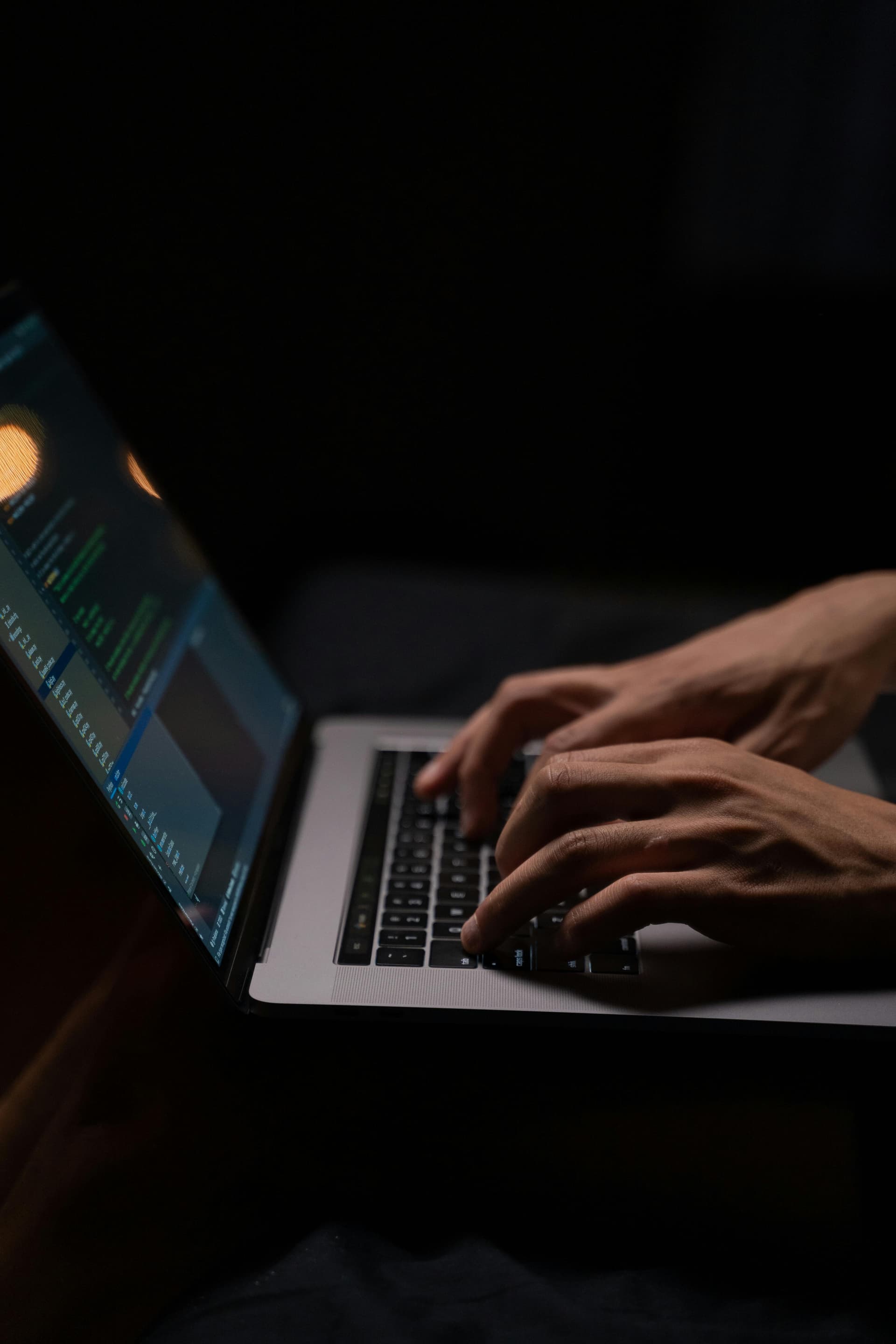
In the hallowed halls of software development, few principles are as universally praised as "good documentation." It's the supposed silver bullet for code maintainability, team onboarding, and technical debt reduction. Yet, as with many silver bullets, there's a dark side that rarely gets discussed: Sometimes, better documentation can actually lead to worse code. Let's explore this heretical idea and understand why it happens – and more importantly, how to prevent it.
The False Promise of Documentation
The Documentation Safety Net
Imagine you're walking a tightrope. Would you be more or less careful if there was a safety net below? This same psychology applies to code and documentation. When developers believe comprehensive documentation will catch any confusion, they often become less rigorous about code quality itself.
This manifests in several ways:
- Complex code justified by detailed explanations
- Architectural decisions obscured by extensive documentation
- Technical debt hidden behind well-written rationales
- Poor naming conventions excused by thorough comments
The Maintainability Mirage
# This function calculates the total price including tax and shippin
# First, it takes the base price and adds state tax (which varies by state)
# Then it calculates shipping based on weight and distance
# Finally, it applies any seasonal discounts
def calc(p, s, w, d, m):
return p * (1 + tax[s]) + (w * d * 0.1) * (1 - (0.2 if m in [6,7,8] else 0))
Sure, the comment explains what's happening. But wouldn't this be better?
def calculate_final_price(base_price, state, weight, distance, month):
tax_rate = get_state_tax_rate(state)
shipping_cost = calculate_shipping_cost(weight, distance)
seasonal_discount = get_seasonal_discount(month)
return base_price * (1 + tax_rate) + shipping_cost * (1 - seasonal_discount)
The second version barely needs documentation because the code itself tells the story. This is the heart of the documentation paradox: Often, the energy spent writing detailed documentation would be better spent making the code more self-documenting.
The Hidden Costs of Over-Documentation
Cognitive Load and Context Switching
When documentation becomes extensive, developers must maintain two parallel mental models:
- The code's actual behavior
- The documentation's description of that behavior
When these diverge – and they always do – it creates cognitive overhead. Each time a developer needs to cross-reference between code and documentation, they pay a context-switching penalty.
The Dual Maintenance Burden
Every line of documentation is a liability that must be maintained. Consider this common scenario:
- Developer A writes code with detailed documentation
- Developer B modifies the code
- Developer B forgets to update the documentation
- Developer C reads the documentation and uses it to write new code
- The system breaks in subtle ways
Now multiply this by hundreds of functions across dozens of developers. The problem becomes exponential.
Documentation as Technical Debt
We often think of documentation as a solution to technical debt, but documentation itself can become a form of debt. Here's how:
- Outdated documentation is worse than no documentation
- Maintaining documentation requires ongoing investment
- Extensive documentation can mask code smells
- Documentation can become a crutch for poor code organization
The Psychology Behind Over-Documentation
The Illusion of Completeness
Comprehensive documentation creates a false sense of security. It's like having a detailed map of the wrong territory – worse than having no map at all because it inspires unwarranted confidence.
The Documentation Theater
Many development teams engage in what might be called "documentation theater" – creating extensive documentation to feel productive or professional, even when that documentation adds little practical value.
Signs you might be engaging in documentation theater:
- Documentation that's written once and never read again
- Documentation that's more complex than the code it describes
- Documentation that exists primarily to satisfy process requirements
- Documentation that makes simple concepts seem complex
Finding the Right Balance
The Documentation Hierarchy
Not all documentation is created equal. Here's a hierarchy from most to least valuable:
- Architecture Decision Records (ADRs)
- Document why, not how
- Focus on decision context
- Capture alternatives considered
- System-Level Documentation
- High-level architecture
- System boundaries
- Integration points
- API Documentation
- Contract specifications
- Usage examples
- Error scenarios
- Code-Level Documentation
- Complex algorithm explanations
- Non-obvious side effects
- Business rule rationales
The Self-Documenting Code Principle
The best documentation is no documentation. Here's how to write self-documenting code:
- Clear Naming
# Bad
def proc_data(d):
pass
# Good
def process_customer_transactions(daily_transactions):
pass
Consistent Structure
# Bad
class Helper:
def do_stuff(self):
pass
# Good
class TransactionProcessor:
def validate_transaction(self):
pass
def process_transaction(self):
pass
def log_transaction(self):
pass
Explicit Interfaces
# Bad
def update(data):
pass
# Good
def update_user_profile(
user_id: int,
new_profile_data: UserProfileData
) -> UpdateResult:
pass
Practical Strategies for Better Documentation
Documentation as Code
Treat documentation like code:
- Keep it DRY (Don't Repeat Yourself)
- Review it during code reviews
- Test it (especially for examples and tutorials)
- Version control it
- Refactor it regularly
The Three-Question Rule
Before writing documentation, ask:
- Could this be made clearer in the code itself?
- Will this documentation likely become outdated?
- What problem am I solving with this documentation?
Documentation Patterns That Work
- The Why, Not How Pattern
# Bad
# This function iterates through the list and counts occurrences
# Good
# We use a Counter instead of a loop for better performance with large datasets
The Context Pattern
# Bad
# Calculate tax
# Good
# Tax calculation follows 2023 EU VAT regulations for digital goods
The Warning Pattern
# Bad
# Process the data
# Good
# WARNING: This operation is not atomic and should be called within a transaction
Tools and Practices for Modern Documentation
Documentation as Tests
Consider property-based testing and documentation:
from hypothesis import given, strategies as st
@given(st.integers(), st.integers())
def test_add_commutative(a, b):
"""
Addition is commutative: a + b == b + a
This test serves as both documentation and verification
"""
assert add(a, b) == add(b, a)
Living Documentation
Implement documentation that stays current:
- Generated API docs from code
- Automated diagram generation from code
- Example validation in CI/CD
- Documentation coverage metrics
The Future of Documentation
AI-Assisted Documentation
As AI tools become more sophisticated, they're changing how we think about documentation:
- Automated documentation generation
- Documentation quality checking
- Natural language queries of codebases
- Real-time documentation updates
Documentation Evolution
The future of documentation might not look like documentation at all:
- Interactive code examples
- Visual programming interfaces
- Self-explaining systems
- Context-aware documentation
Conclusion: A New Documentation Philosophy
The solution to the documentation paradox isn't to stop writing documentation – it's to write better code that needs less documentation. Here's a framework for modern documentation:
- Make your code as self-documenting as possible
- Document the why, not the how
- Keep documentation close to the code
- Automate what can be automated
- Regular documentation reviews and cleanup
- Focus on high-value documentation types
Remember: The goal isn't to write perfect documentation; it's to build maintainable systems. Sometimes that means writing less documentation and spending more time on code quality.
As you evaluate your next documentation task, ask yourself: "Am I documenting this because the code isn't clear enough?" If the answer is yes, consider refactoring first. Your future self (and your teammates) will thank you.
Related Posts
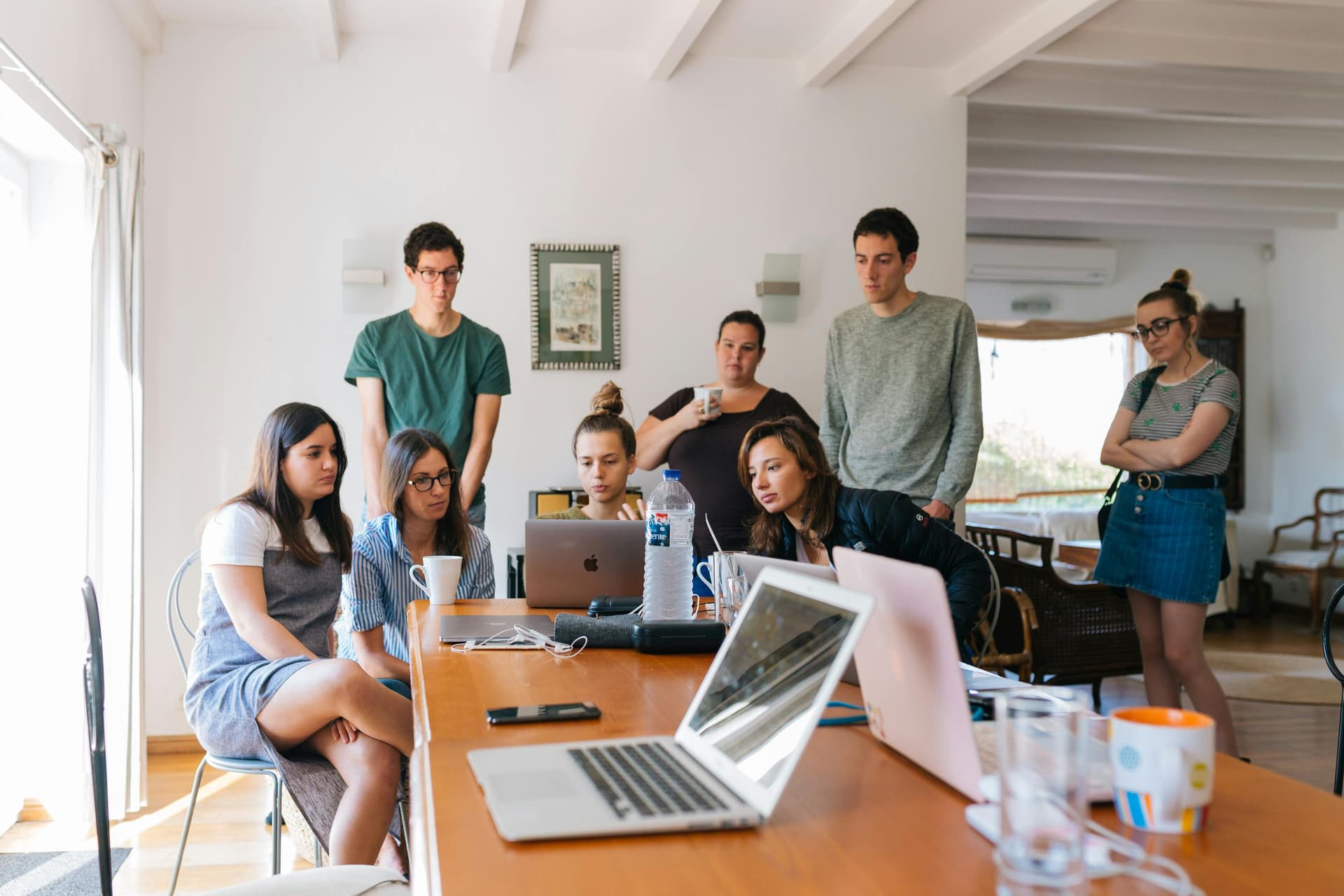
How to Launch a Tech Startup in 2025: Complete Founder's Guide
Starting a tech startup? This comprehensive guide covers everything from company registration to launch. Learn how to build a strong foundation and avoid common pitfalls.
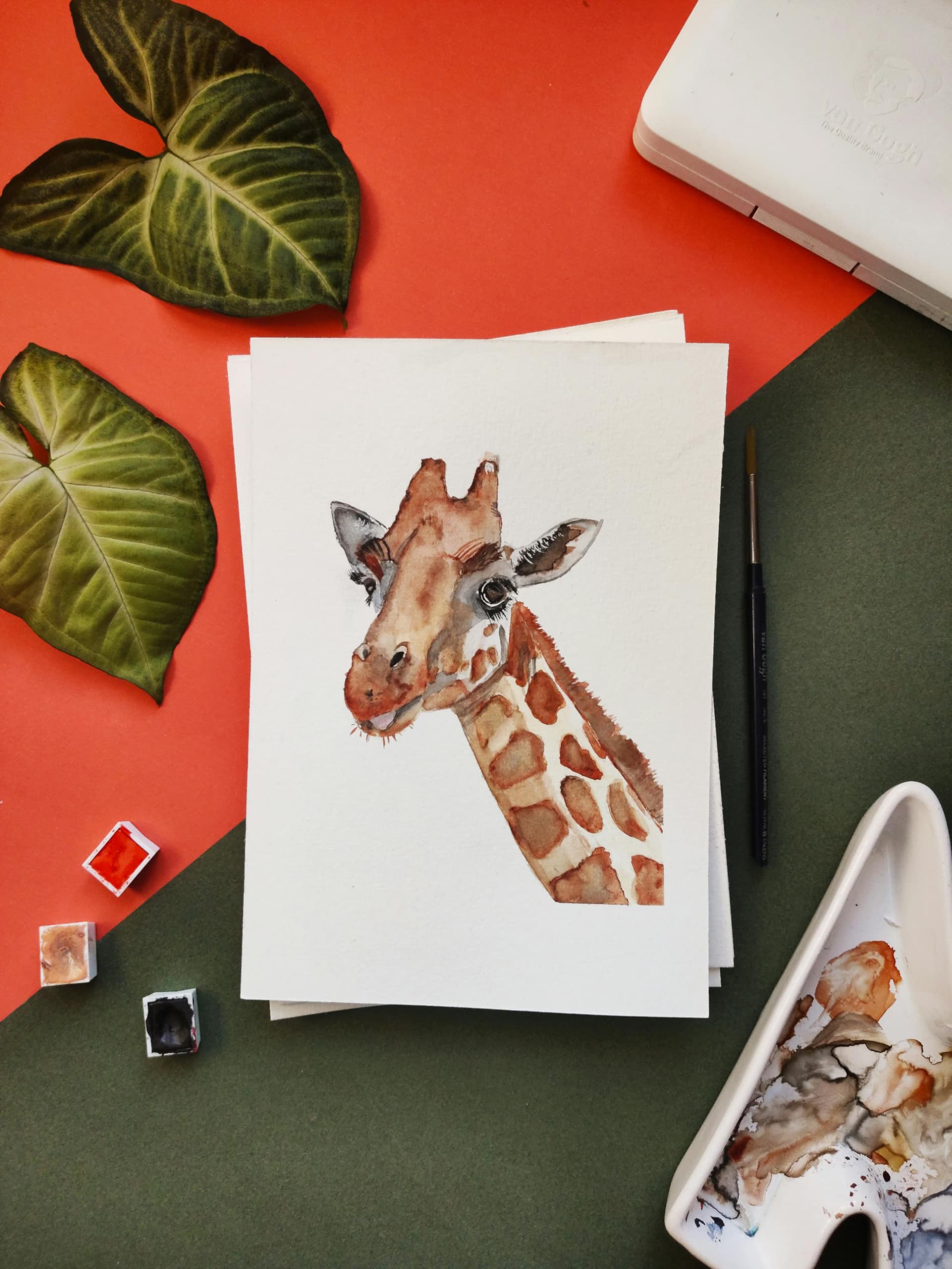
I Got Fired Over AI-Generated Work (And It Wasn't What You Think)
Everyone talks about AI replacing jobs, but nobody mentions this hidden danger of AI in the workplace. Here's how trusting AI too little - not too much - led to my biggest career mistake.
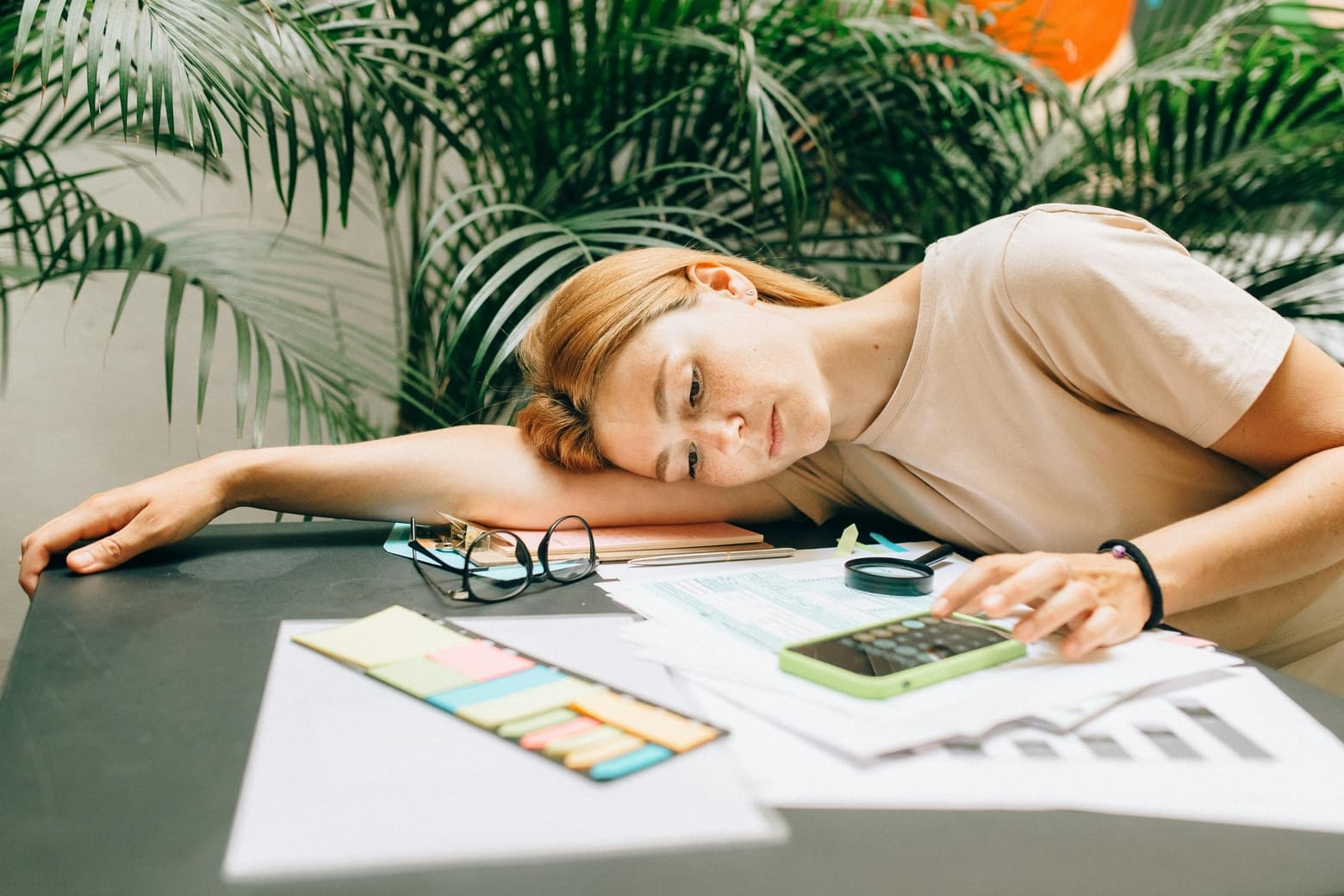
The 'Task Debt' Crisis: How Unfinished Side Projects Are Actually Making You a Better Developer
Every developer has that folder of half-finished projects gathering digital dust. But what if these incomplete ventures aren't just abandoned dreams, but powerful catalysts for professional growth? Here's how your 'task debt' is secretly making you a better programmer.
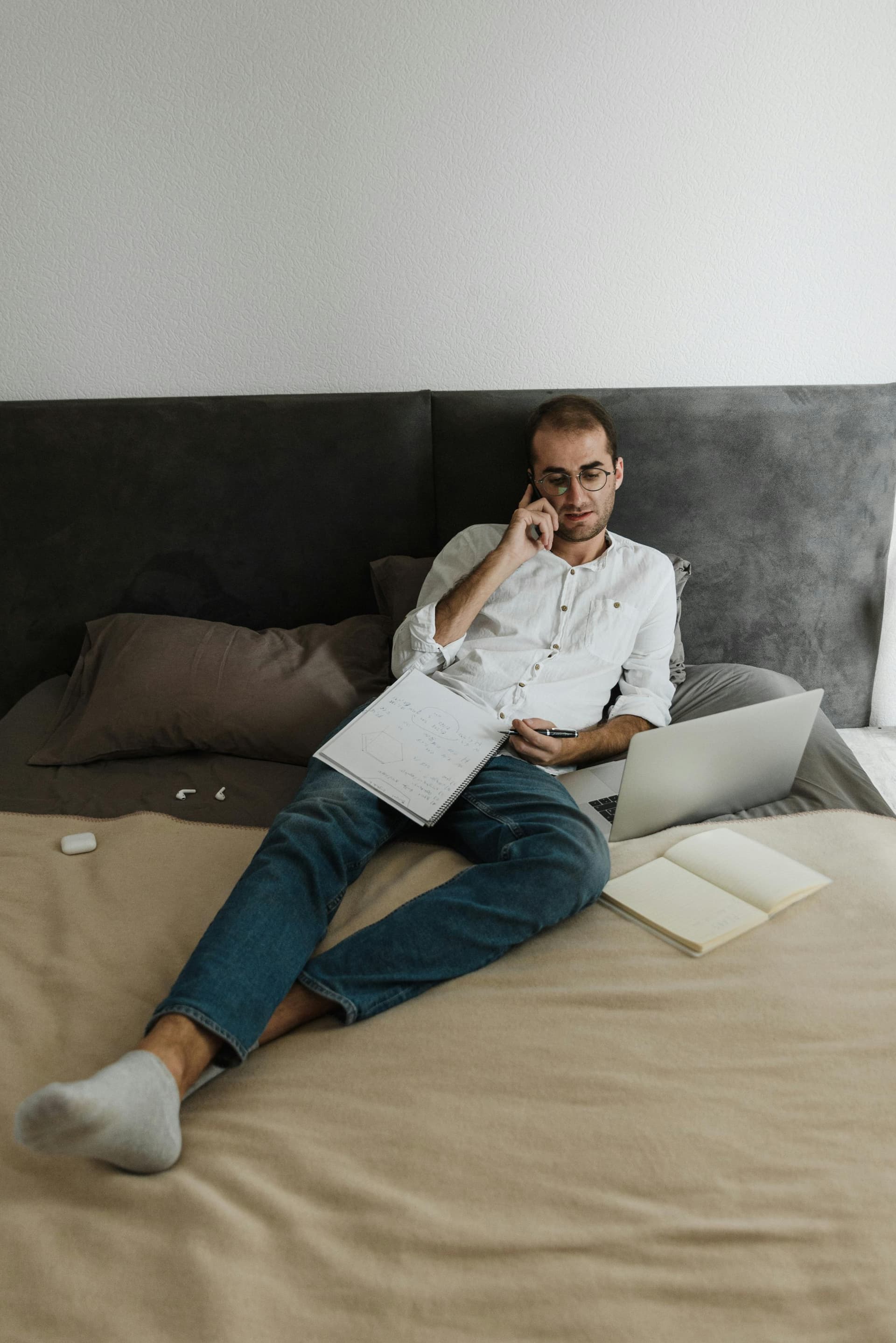
Remote Work Made Engineers Worse (The Data Nobody Wants to Share)
When Microsoft noticed their engineers' code quality dropping 23% post-remote, they buried the report. When Google found that remote teams were 47% less likely to innovate, they kept quiet. Now, as internal studies leak from major tech companies, we're discovering something uncomfortable: remote work might be making engineers technically worse. Not because they're working less, but because they're learning less. And the implications are starting to worry tech leaders.
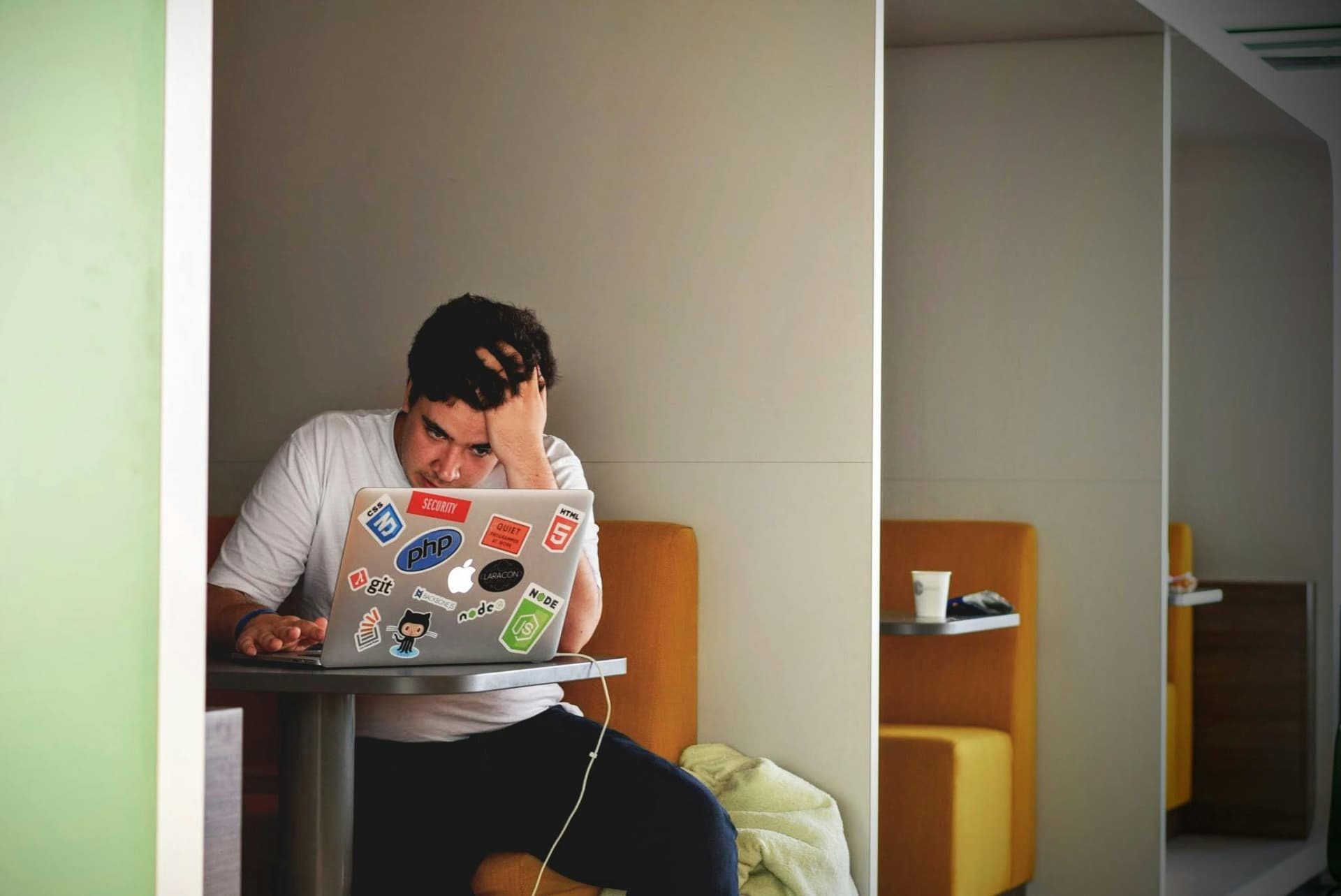
Why Bad Programmers Will Survive The AI Revolution (And Good Ones Should Worry)
When Google analyzed which engineers were thriving with AI tools, they found something disturbing: their "average" programmers were outperforming their technical experts. The reason? Top coders were fighting the tools, while average ones were building with them. Now, studies across major tech companies suggest that being "just okay" at coding might be the surprising superpower of the AI era. And the implications are making tech leaders nervous.
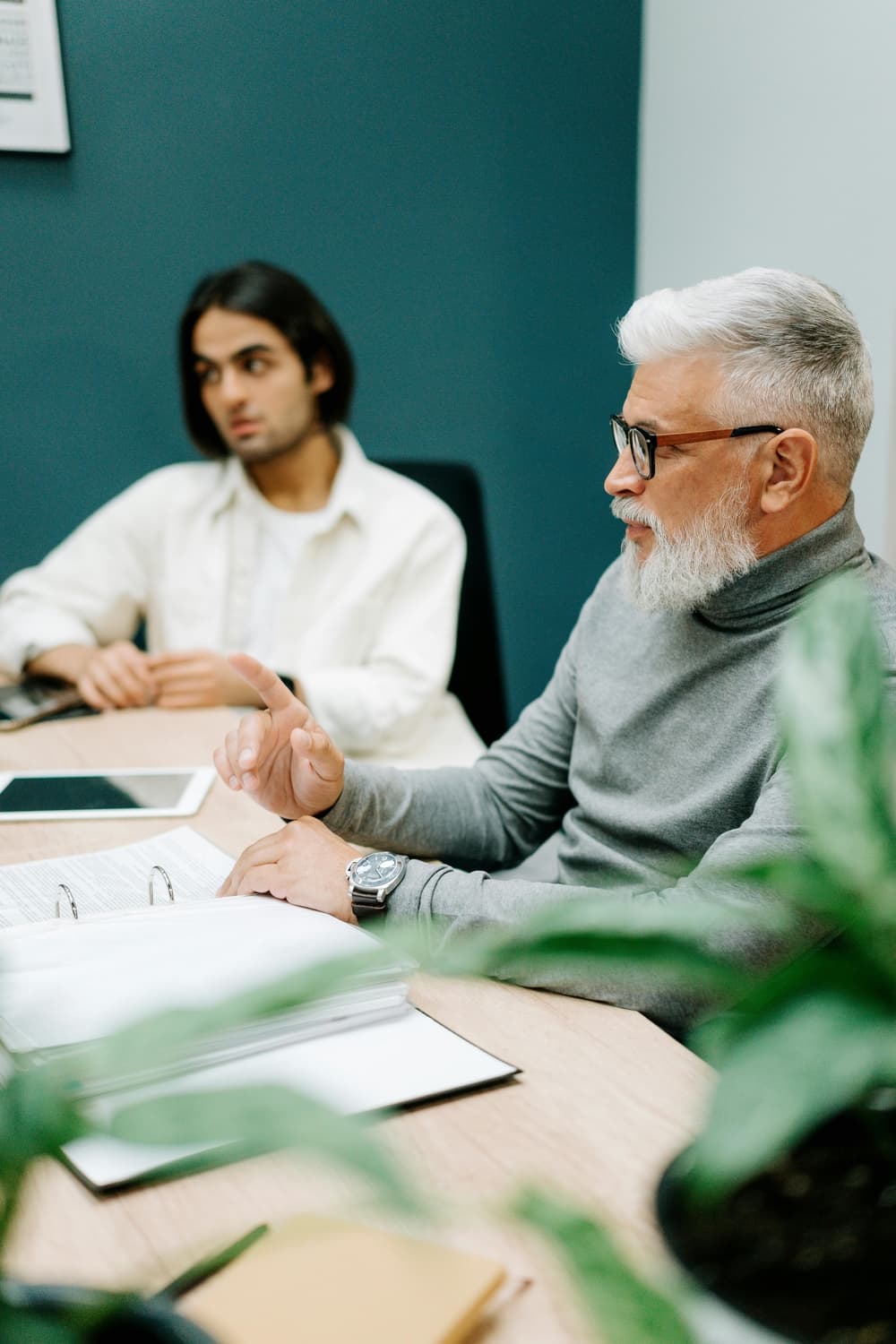
Junior Developers Are Making Seniors Obsolete (Just Not How You Think)
When Amazon discovered their newest hires were outperforming veterans in AI integration, they looked for coding expertise differences. Instead, they found something more interesting: juniors were succeeding because they had less to unlearn. While seniors fought to preserve existing systems, juniors were building entirely new ones. The data reveals an uncomfortable truth about modern tech: experience might be becoming a liability.
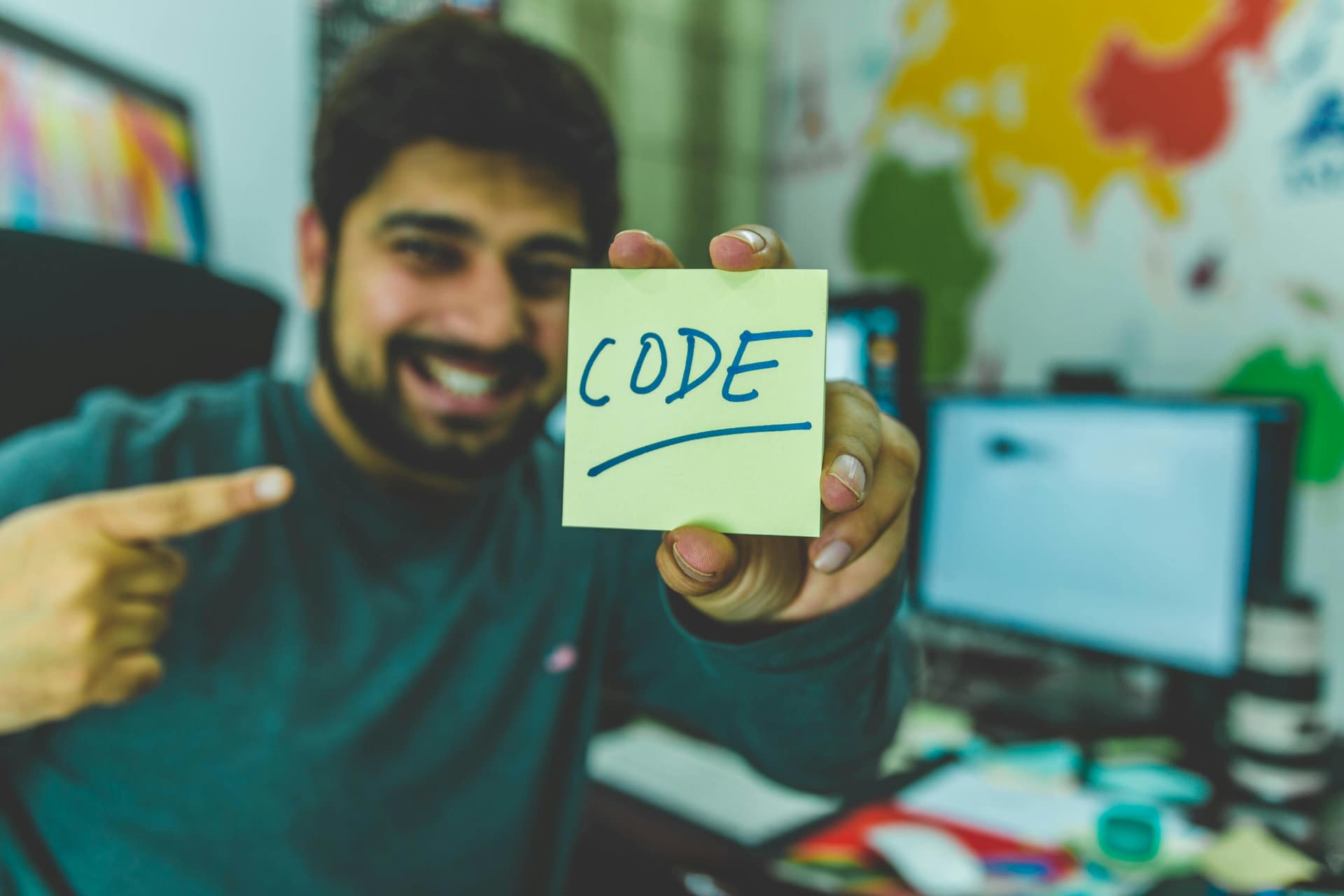
The Most Valuable Programming Language is English
When Microsoft traced their failed projects back to root causes, poor code ranked sixth. Poor communication ranked first. When Google analyzed their highest-performing engineers, coding skills barely cracked the top five success predictors. The highest correlation with success? Written communication ability. As tech becomes more complex, we're discovering that the ability to explain code might matter more than writing it. And the data is starting to make programming language wars look pointless.
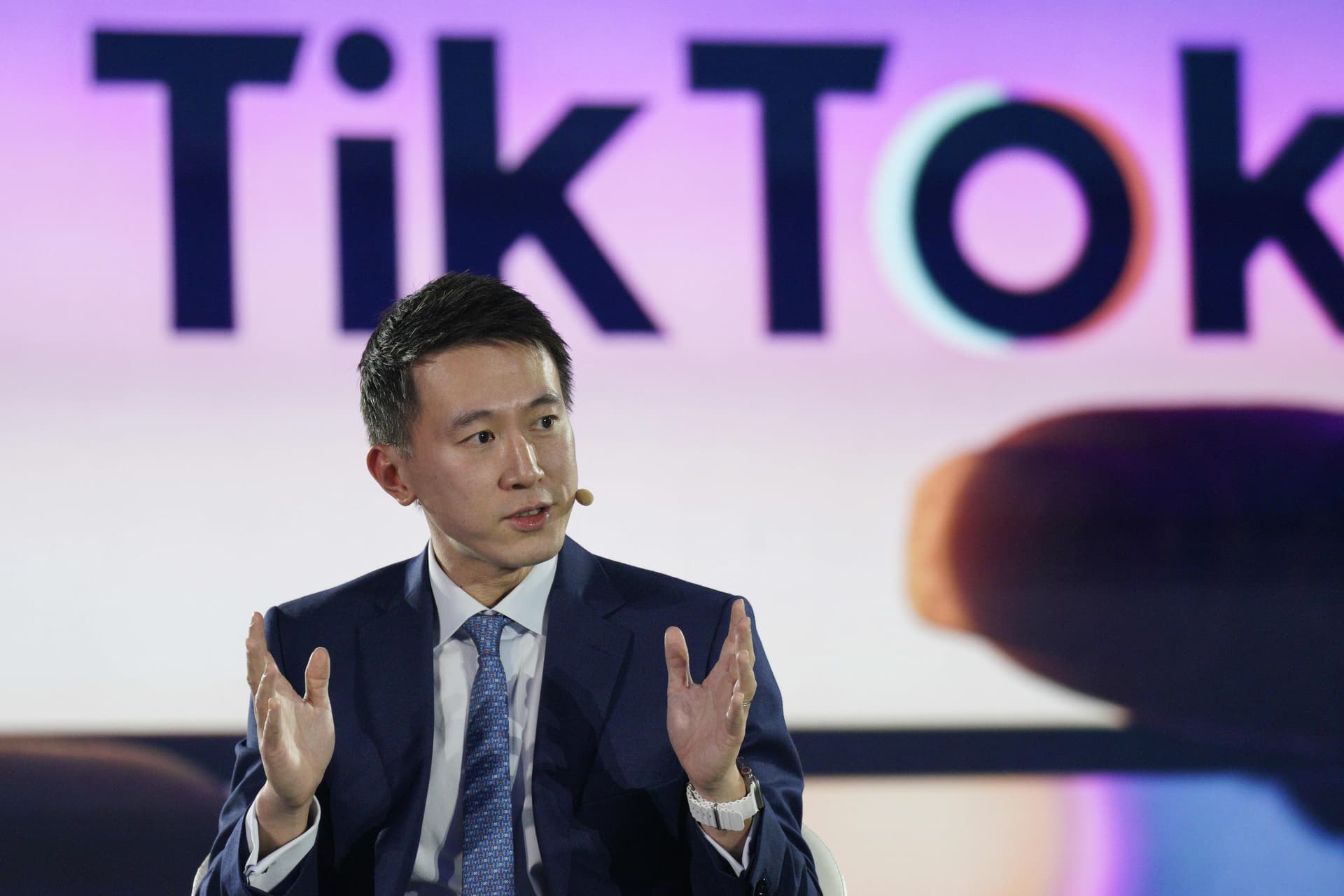
TikTok's Hidden EdTech Empire: The Accidental Learning Revolution
When MIT researchers discovered that engineering students were learning advanced manufacturing concepts faster on TikTok than in lectures, they dismissed it as an anomaly. Then Harvard's EdTech lab found similar patterns in medical education. Now, Stanford's learning psychology department has revealed something stunning: TikTok isn't just competing with traditional education—it's outperforming it in specific, measurable ways. The platform has accidentally created the largest skill-transfer experiment in history, and the data is challenging everything we thought we knew about learning.
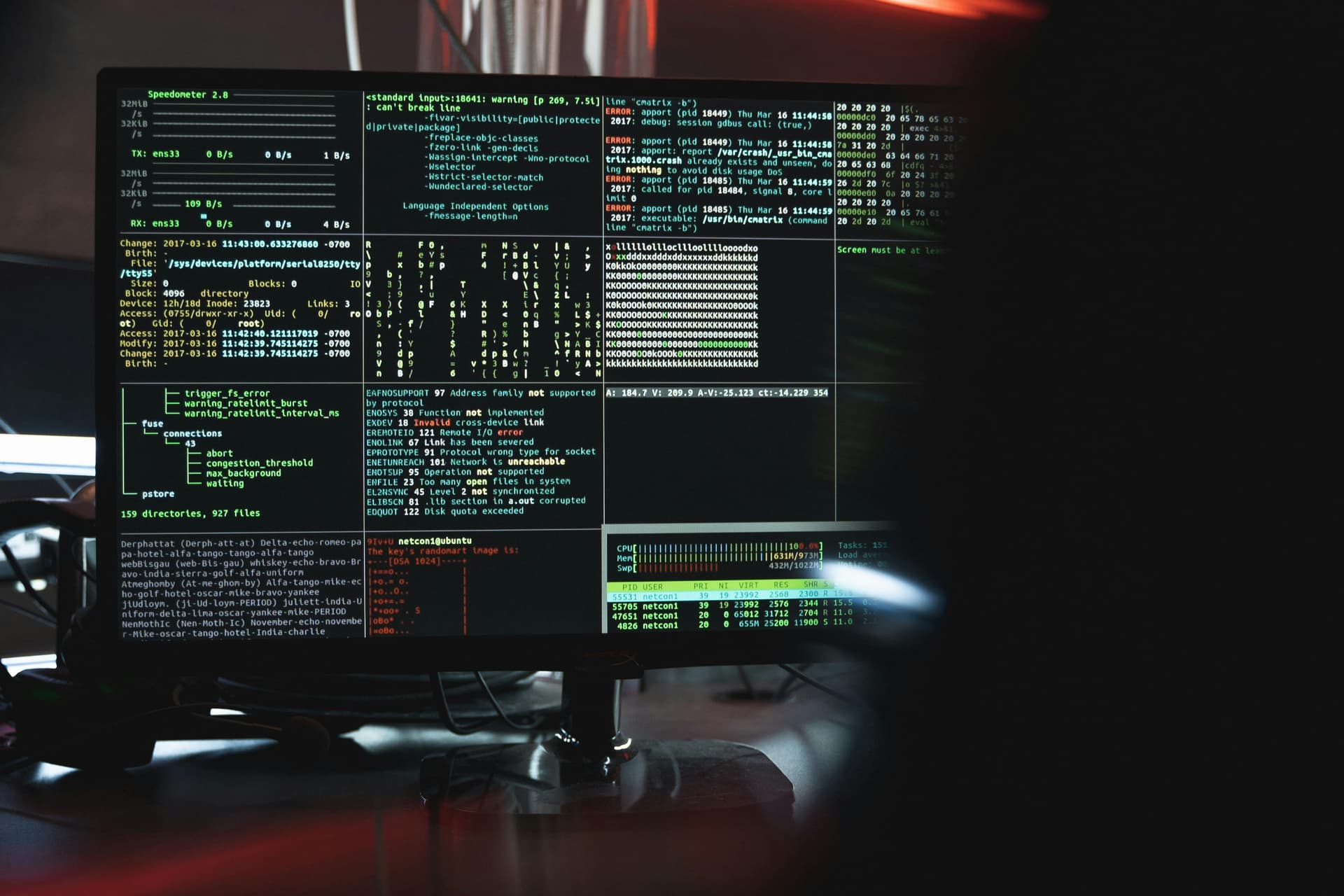
The Terminal is Dead: Why Senior Developers Are Abandoning the Command Line
When Linus Torvalds casually mentioned he spends 80% less time in the terminal than five years ago, Linux zealots demanded an explanation. His response? "Modern development isn't about typing speed anymore." GitHub's internal data tells an uncomfortable story. Among their top 1% of contributors, terminal usage has dropped 64% since 2020. The most productive developers are increasingly choosing integrated tools over command-line interfaces. And they're shipping more code than ever.
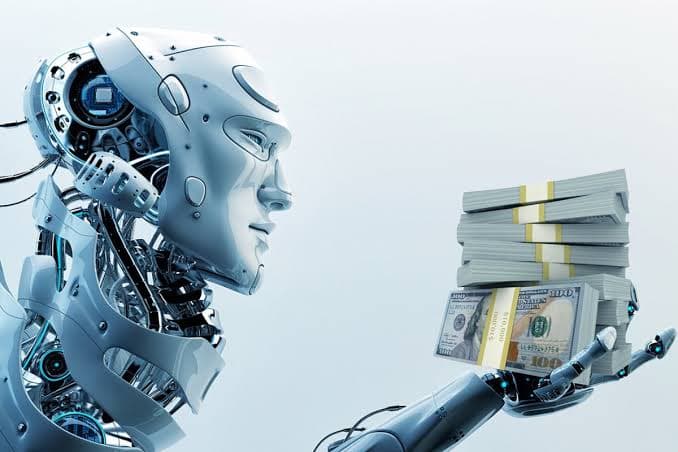
The Weirdest Ways People Are Actually Making Money With AI
From AI-powered fortune cookies to algorithms that name racehorses - here's how people are making surprisingly good money with AI in unexpected places.
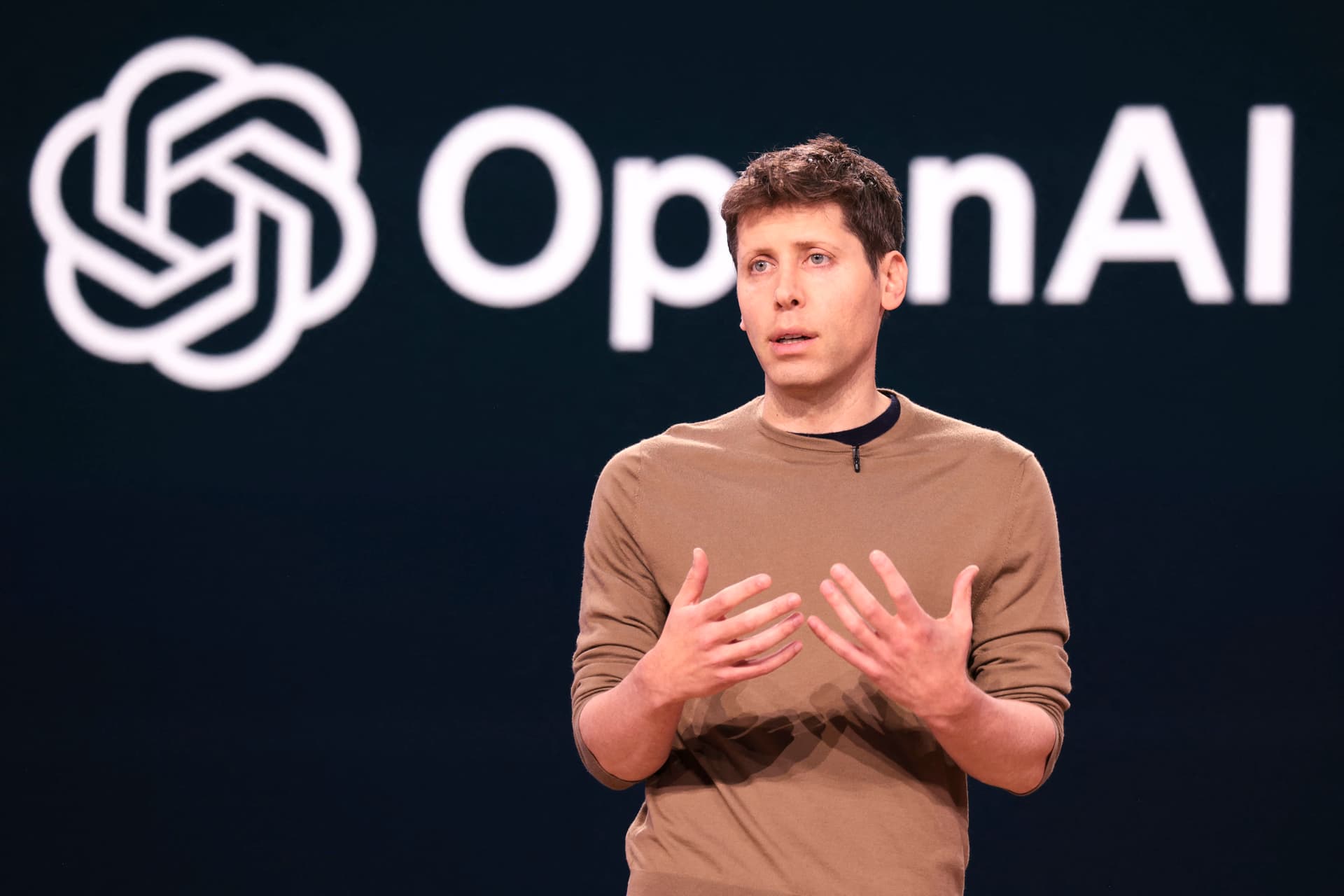
Everyone Missed These AI Startup Gaps
Forget chatbots. Here's where AI startup opportunities actually exist, from niche market needs to overlooked industry pain points
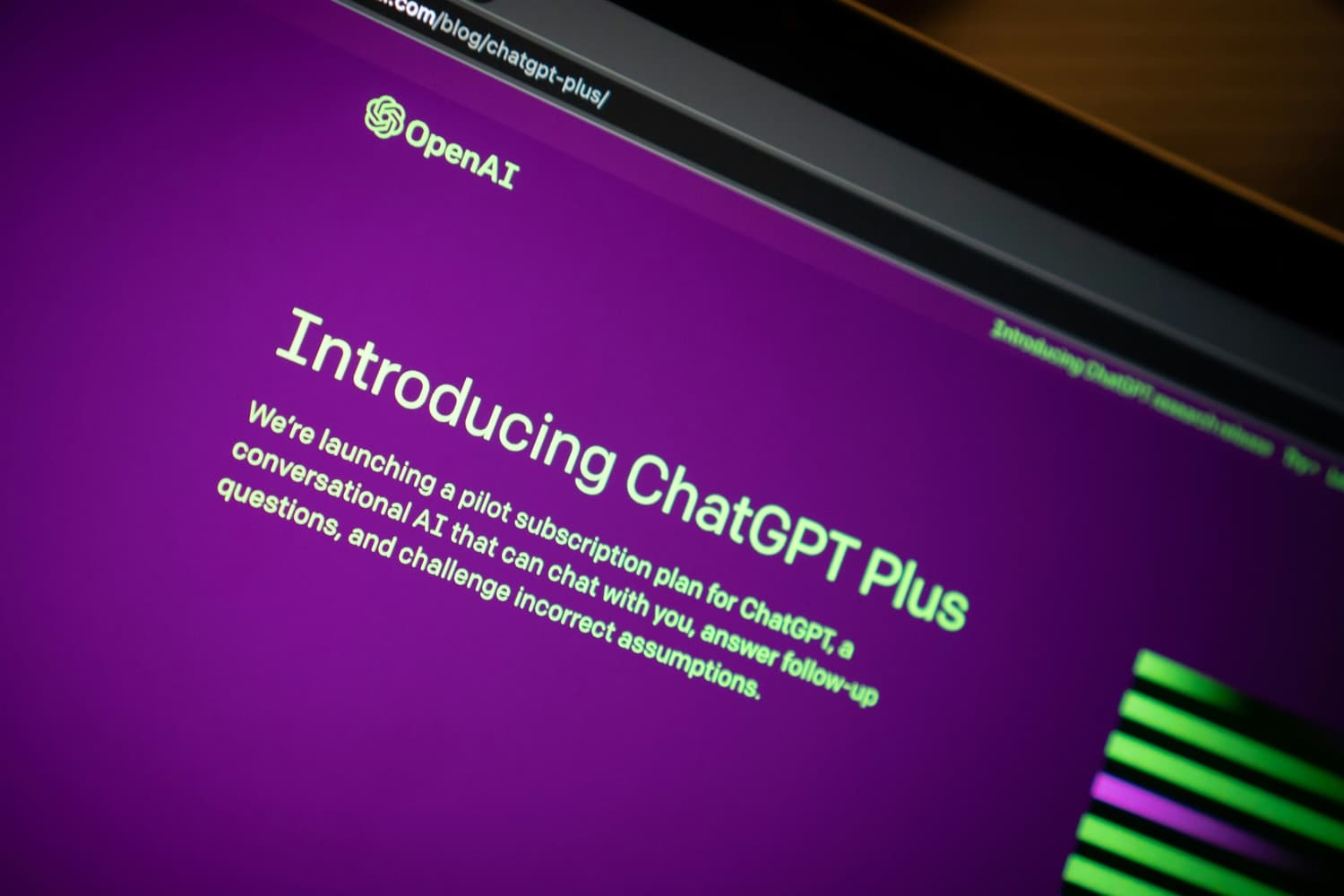
Why ChatGPT Gives Your Parents Better Answers Than You
AI models respond differently to different age groups. Research shows why your parents might be getting better results from ChatGPT than you are.
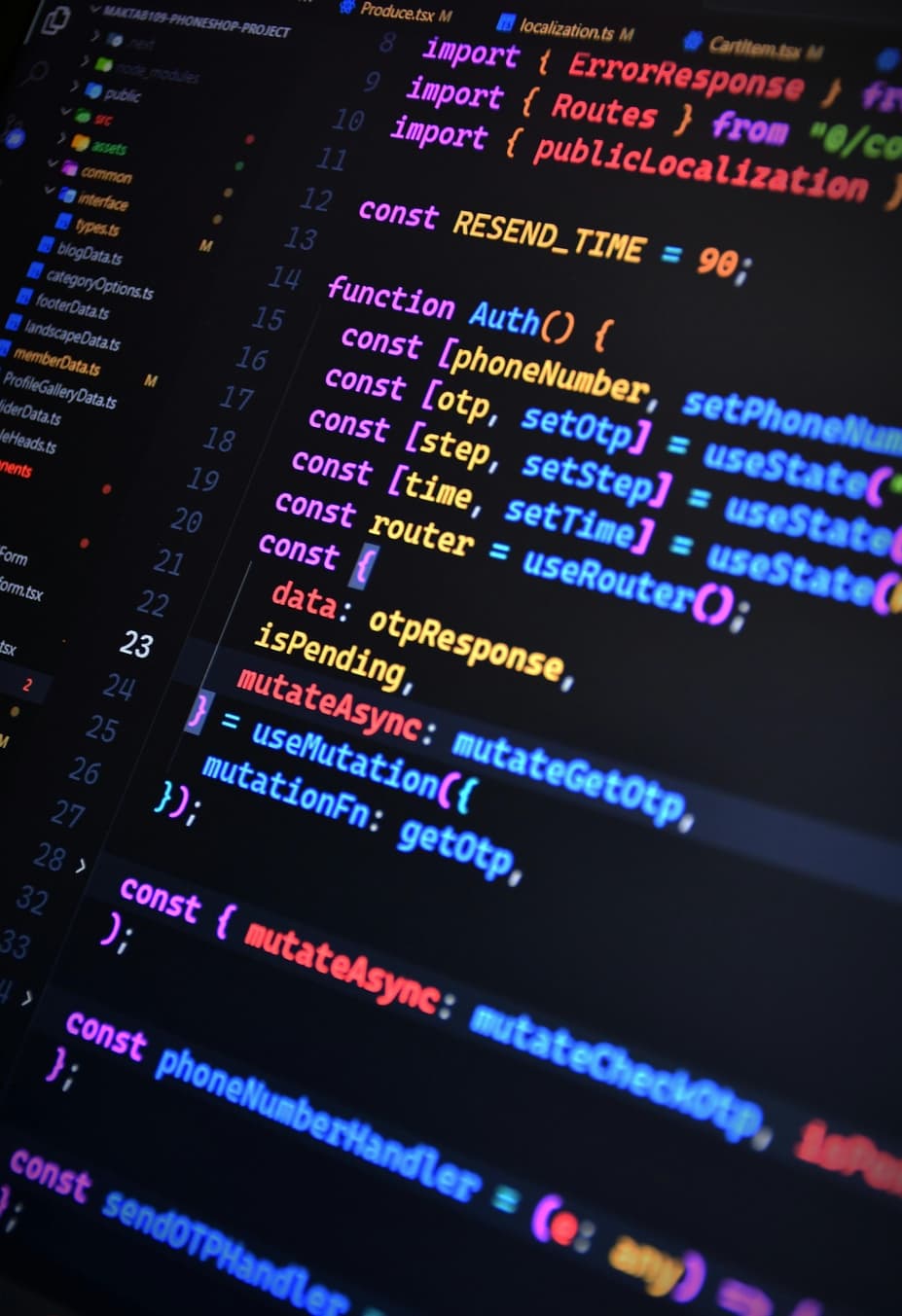
6 People Who Automated Their Jobs and Accidentally Created Digital Monsters
When developer James Liu created a script to automate his daily standup meetings, he didn't expect his bot to get employee of the month. When marketer Sarah Chen automated her social media, she didn't plan for her bot to start a Twitter war with Elon Musk. Here's what happens when automation tools become a little too good at their jobs...
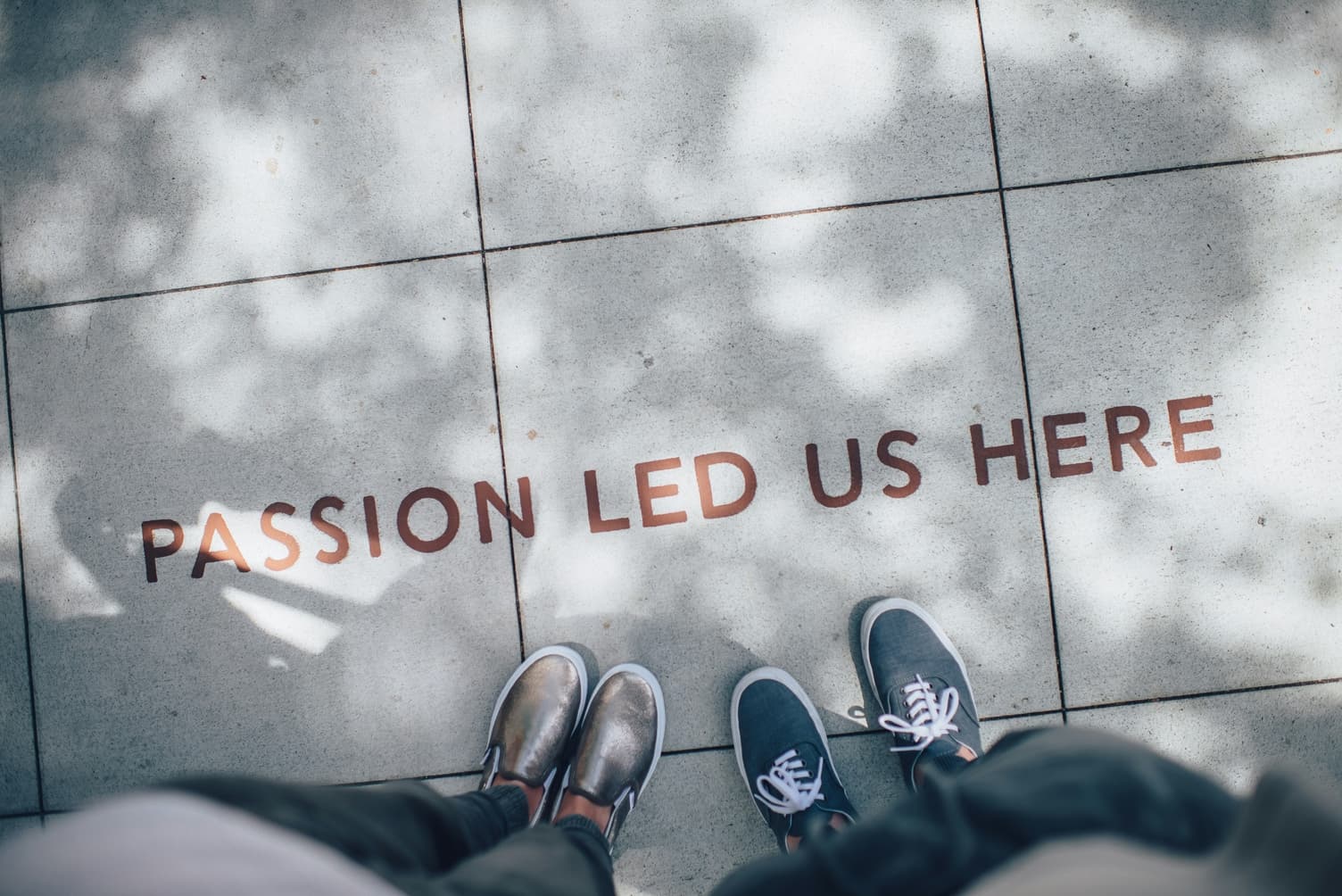
The Pull Request That Changed Everything: A Developer's Journey from Code to Leadership
It was 2:47 AM when Maya finally pushed her code. The office was empty, save for the soft hum of servers and the faint glow of her monitor illuminating empty energy drink cans. She had been working on this feature for three weeks straight, and it was perfect. Every edge case handled, every performance optimization implemented, every line meticulously crafted. The pull request was massive – 2,847 lines changed across 23 files. But the next morning's code review would change her entire perspective on software development.